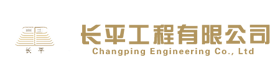

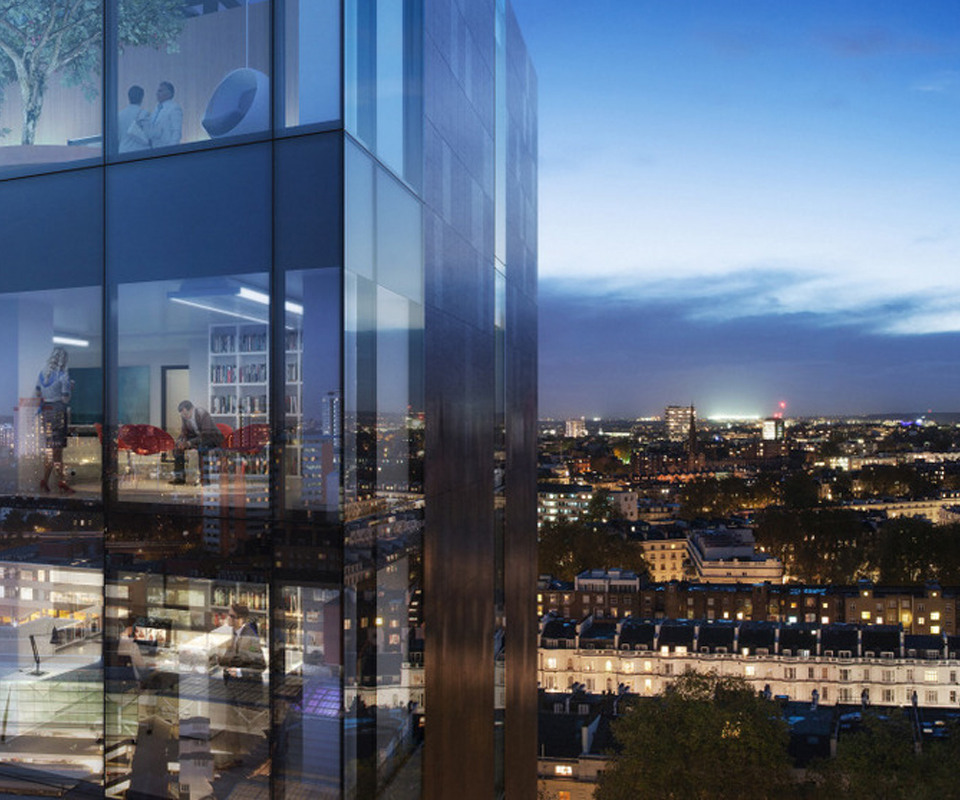
公司简介 ABOUT US
——————————————
开云app登录入口(中国)官方网站
开云app登录入口(中国)官方网站(Changping Engineering Co.,ltd)成立于2005年7月,办公地址位于太原市杏花岭区马道坡街东一号院长华湾小区六号楼一层东垮二号,注册资金5000万元。公司拥有工程勘察专业类(工程测量、开云app登录入口(中国)官方网站(勘察,物探测试检测监测))甲级资质;检验检测机构资质;工程质量检测机构资质:人工地基工程检测专项资质;地基基础工程专业承包壹级资质、特种工程(建筑物纠偏和平移)专业承包资质、特种工程(特种防雷)专业承包资质;地震安全性评价从业资格;工程勘察专业类(开云app登录入口(中国)官方网站(设计)、水文地质勘察)乙级资质;工程勘察工程钻探劳务资质;工程测绘乙级资质;地质灾害评估和治理工程勘查设计乙级资质;地质灾害治理工程施工资质乙级;环保工程专业承包叁级资质。
0
成立15余载
0
7大服务项目
0
技术人员40余人
0
1300+工程项目
技术规范 品质优良 不断改善 满足客户
Technical specifications, excellent quality, continuous improvement, to satisfy customers
技术雄厚
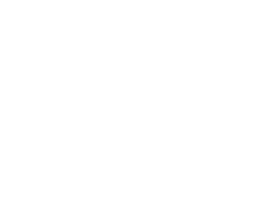
拥有一支理论水平高、实践经验丰富、技术力量雄厚、敢打硬仗的专业化新型队伍
业务范围广
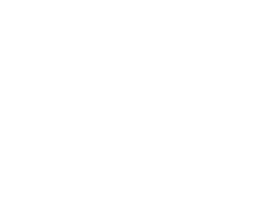
业务范围涉及工民建、市政建设、工矿企业、交通、能源等领域
设备齐全
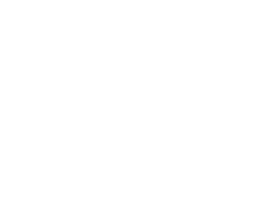
公司技术装备配套齐全, 均为国内科技领先的专业生产及施工设备
管理体系完善
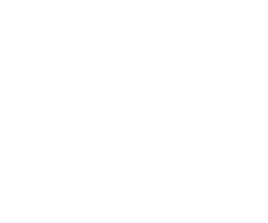
公司坚持诚信经营、创造精品、用户满意,竭诚为社会提供优质服务的理念
联系我们
CONTACT US
电话:0351-4670078
手机:13333510360 / 15935131061
微信:13333510360/tycpyt
邮箱:tycpyt@163.com
地址:太原市杏花岭区马道坡街东一号院/长华湾小区六号楼一层东垮二号(杨家峪高速口西200米路南)
手机:13333510360 / 15935131061
微信:13333510360/tycpyt
邮箱:tycpyt@163.com
地址:太原市杏花岭区马道坡街东一号院/长华湾小区六号楼一层东垮二号(杨家峪高速口西200米路南)
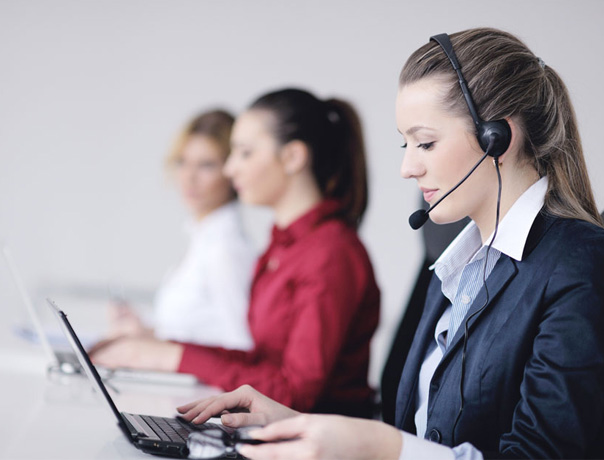
©2021 开云app登录入口(中国)官方网站 晋ICP备17010111号-1 技术支持 - 资海科技集团
©2021 开云app登录入口(中国)官方网站 晋ICP备17010111号-1 技术支持 - 资海科技集团